Post Archive for 2016
As promised, here's a sequel post for creating a simple 'Hello World' console app in C++ for your Oculus Rift, this time covering the final production 1.3.0/1.3.2 Oculus SDK for CV1 and DK2 devices.
If you've not yet set up your environment for C++ development, the earlier Oculus Hello World post for the v0.5.0 runtime will give you the steps you're wanting to follow for that.
The latest Oculus SDK can be retrieved from here:
https://developer.oculus.com/downloads/With the Oculus v1.3.2 runtime provided through the general Oculus setup program, here:
https://www.oculus.com/en-us/setup/For the code itself, this simple program:
#include <iostream>
#include <iomanip>
#include <OVR_CAPI.h>
#include <thread>
#define COLW setw(15)
using namespace std;
int main()
{ // Initialize our session with the Oculus HMD.
if (ovr_Initialize(nullptr) == ovrSuccess)
{
ovrSession session = nullptr;
ovrGraphicsLuid luid;
ovrResult result = ovr_Create(&session, &luid);
if (result == ovrSuccess)
{ // Then we're connected to an HMD!
// Let's take a look at some orientation data.
ovrTrackingState ts;
while (true)
{
ts = ovr_GetTrackingState(session, 0, true);
ovrPoseStatef tempHeadPose = ts.HeadPose;
ovrPosef tempPose = tempHeadPose.ThePose;
ovrQuatf tempOrient = tempPose.Orientation;
cout << "Orientation (x,y,z): " << COLW << tempOrient.x << ","
<< COLW << tempOrient.y << "," << COLW << tempOrient.z
<< endl;
// Wait a bit to let us actually read stuff.
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
ovr_Destroy(session);
}
ovr_Shutdown();
// If we've fallen through to this point, the HMD is no longer
// connected.
}
return 0;
}
Will connect to your Oculus HMD (Head Mounted Display) using the Oculus 1.3.2 runtime, read the device's orientation and output that data to console, with the code structure essentially the same as for the previous v0.5.0 SDK example
The earlier Oculus 'Hello World' example connected directly to the device's raw sensor data, but that option is no longer being provided under the Oculus 1.3.2 SDK. Unfortunately, that's not the only limitation presented by the Production Oculus SDK.
If you enjoyed writing C++ programs for the Oculus DK2, I have some bad news for you.
The Bad News for C++ Development on Oculus
When starting the simple "Hello World" example provided above on your Oculus CV1 or DK2, you'll notice two things.
First, the Oculus Store opens:
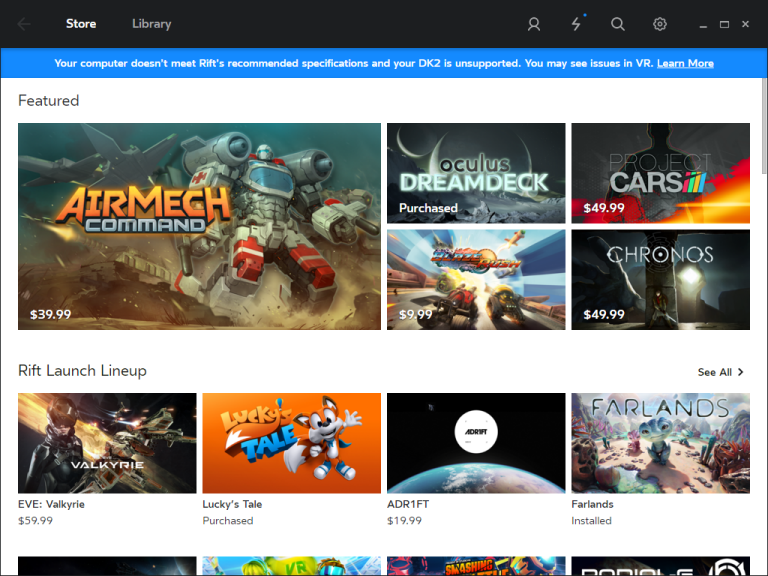
Yes, that happens every time you connect to the Oculus, no matter how trivial your program is.
No, you cannot do anything to turn that off.
It's not an exaggeration to call this horrible: It's as if Logitech or Microsoft had set up a store to be shown on your screen whenever you plugged in your mouse and provided no means at all to be able to dismiss it.
It gets worse.
The second thing you'll notice is that your HMD displays a Health and Safety warning which occupies your Oculus screen until dismissed. You can dismiss that either by positioning a cursor on-screen for a few moments or clicking the "A" button on your Oculus XBox controller.
If you're developing software, that rhythm gets really old, really fast and, no, Oculus provides no mechanism for bypassing it.
Finally, after dismissing that warning, your HMD will display a "Please Wait..." message and, if you're looking to develop a simple console app to run alongside your Oculus, that's where things stop.
The Really Bad News for C++ Development on Oculus
Unfortunately, it appears the Oculus v1.3.2 runtime no longer allows console applications to run in parallel with apps on the HMD.
Even the trivial program shown above cannot run at the same time as an Oculus game or movie: If you start a console exe which connects to your Oculus HMD, any other active Oculus app will freeze and your HMD will be held at the "Please Wait..." screen until your exe is halted.
That might change in the future, with the blocking of external console apps hopefully being a temporary bug in the system rather than an obstruction put in place by Oculus by design but, for now at least, general C++ hacking with the Oculus Rift seems to have come to an end.
That's perfectly fine if you just want to play games and watch movies on your HMD but, if you're a developer who likes to play with their computer equipment and dream up options for parallel programs running alongside your apps, casual development on Oculus has now been stopped in its tracks.
Oculus No Longer a Developer-Friendly Tool
Unlike the DK2 we enjoyed hacking around with under the beta SDKs, the Oculus CV1 no long provides a nifty bundle of sensors we can interact with. Oculus now seems set to become a locked-down 'social experience' which exists to sell you stuff and track how you interact with your friends.
The terms of service for the Oculus are notoriously creepy with no promises that user data and activity history will be kept from targeted advertising in the future.
It's a little sleazy.
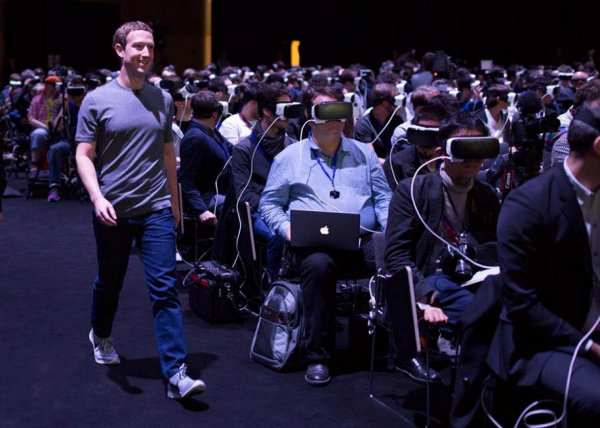
And more than a little disappointing.
The Oculus DK2 was fun and satisfying to develop with, but the production Oculus toolkit just isn't for me.
If you're wanting to build an app for Oculus, I would recommend sticking with a game development environment like Unity3D or Unreal Engine, as hacking around in C++ with the sensors and other nifty hardware bits seems likely to bring you frustration and pain with the current Oculus development tools.
For fun hacking around of that sort, it seems like it's time to turn to the HTC Vive and Open VR.
So, you have a C++ program with a Qt interface for Windows that you're releasing as a quick 'Version One'.
All is well and you're ready to ship... until you discover that DPI scaling in Windows resizes all label text by 125%, 150% or 200% while leaving buttons and labels at their set dimensions. The result with that scaling is an interface which is messy at best and unreadable at worst.
"Hey, no problem," you're thinking. "I'll just set Qt to ignore the Windows DPI setting and have the app drawn as we created it.
"Sure, the text will be smaller if the user is running Windows with a font scaled at 125% or 150% above normal, but everything will remain legible and laid out correctly in our app. We can revisit the GUI to do everything correctly later."
If this is a familiar tale for you, you already know there is no flag for setting Qt to ignore the Windows DPI setting.
What? Seriously?
You've seen the forum posts chiding that a proper Qt GUI should use a scalable layout. You've read the smarmy comments claiming that slapping together a new GUI with layouts should be easy for an experienced Qt developer to do. So easy, in fact, that the lack of documentation on the subject and complete absence of examples should be no deterrent to a Qt developer looking to create a layout capable of automatic scaling with Windows fonts.
Gee, thanks.
Give Me a Qt Quick Fix for High-DPI Displays Already!
Sure, rewriting that 'Version One' Qt GUI with scalable layouts would be awesome and all, but I'm new to Qt, have a hard release deadline, no time, and the number of users currently affected by the issue can be counted on one badly mutilated hand.
A full GUI re-write for an app which might end up discarded is the least responsible thing to do right now. Isn't there a way to tell Qt "Don't scale text to accommodate high-DPI displays"?
Why, yes there is! You can explicitly set the size of fonts in your Qt app with font.setPixelSize.
For example, this:
QApplication a(argc, argv);
QFont font = qApp->font();
font.setPixelSize(11);
qApp->setFont(font);
Will size all GUI fonts at 11 pixels in size regardless of the DPI settings on Windows.
It's simple, it's an imperfect solution, and it works.
Done. Ship it.
We can look at a proper font scaling solution and rebuild the GUI with Qt's DPI scalable layout magic after we've confirmed the app's Version One satisfies a genuine need.
[NOPE! No longer! Stay Away!]
June 2022 Update:
Microsoft Ajax Minifier is no longer being maintained. Updates halted in 2019.
Instead, I recommend using Uglify.js
Uglify?
Yup!
Uglify's command line tool has improved. I've been able to use the uglifyjs mangle command from a Windows batch file without issue.
Example usage would be:
uglifyjs unobfuscated_code.js --mangle eval=true -o obfuscated_code.js --verbose --warn
Of note: the eval=true option will mangle/rename function and variable names if eval statements are present. The presence of eval statements caused my own function and variable names to not mangle until that option was added.
If you need to switch from ajaxmin.exe, Uglify would be a good choice.
Can I still use Microsoft Ajax Minifier?
The ajaxmin.exe program still works. However, ajaxmin.exe does not properly parse async JavaScript functions.
The moment you add asynchronous JavaScript functions or Promises, Microsoft Ajax Minifier will not work for you.
To save yourself unpleasant surprises when preparing an app build for deployment, I would recommend you switch from ajaxmin.exe at your earliest convenience.
The original blog post remains below, but is no longer accurate.
I recommend using Uglify.js rather than Microsoft Ajax Minifier.
When I started writing JavaScript for production use, the final step in the process was selecting a minifier to wrap the code up to a tidy, ready-to-deploy bundle. Back in the day, the go-to for that was YUICompressor, but times have changed.
Goodbye YUICompressor
I've been using the YUICompressor JavaScript minifier for ages and it was time to update to a more current version of the tool.
Checking out the options, it turns out that YUICompressor (along with all other YUI tools) is no longer being supported by Yahoo as of August 2014. Not a good sign, but it seems the tool is still being supported by a third party so it should be good to go.
Giving the latest YUICompressor 2.4.8 a try, I was immediately presented with an error indicating that the tool could not find the source file to compress. A bit of searching showed me that this particular YUICompressor error in finding the input file's path has been outstanding since 2013 and appears unlikely to be addressed soon.
Hey, we all accidentally deploy bugs every now and then, but when something basic/essential like the input file path for a command line tool remains broken for over three years... Well, nature always finds a way to let us know when software is no longer being supported, and red flags like this are one of those little hints.
With that, it's time to say goodbye to YUICompressor and find another minifier.
So... Uglify?
An online search will quickly show that the go-to JavaScript minifier these days is Uglify.
To be pedantic it's 'Uglify2' (support for the original Uglify has been dropped) but everyone refers to the tool without the appended version number. Web searches for questions about 'Uglify' will usually direct you appropriately, but you'll want to watch out for confusion between 'Uglify' and 'Uglify2' when downloading: The original (abandoned) Uglify is still listed on GitHub.
Uglify works with NodeJS so, if you're like me and had not previously touched NodeJS, a quick detour is required to first install Node and get familiar with the basics before proceeding. This StackOverflow post will provide you with a bit of extra guidance on that if you're as new to NodeJS as I was.
Once NodeJS has been installed, the latest uglify-js package has been retrieved, and your computer has been rebooted to ensure all path variables are set for NodeJS, a run on the Windows command line:
uglifyjs -o destfile.js sourcefile.js
will provide you with a basic minification, with spaces, comments and carriage returns snipped out of the code. Function and variable names remain unminified.
The 'mangle' option in Uglify will apparently handle the minification of variable and function names, but I could not get that working for the life of me.
That seems to be a pretty common issue with Uglify, and some developers have been able to resolve those Uglify function/variable name minification difficulties, but I wasn't one of them. Try as I might, I could not find the magic invocation syntax on Uglify which would bring about full code minification, and the complete lack of use-case examples or even error output at the command line brought me nothing but frustration.
[June 2022 update: Uglify.js was not mangling my function and variable names due to eval statements being present. Setting Uglify to use the "--mangle eval=true" option resolved that for me]
So, even though Uglify seems to be awesome for almost everyone else out there, it just wasn't the tool for me. Time to look elsewhere.
Chosen Solution: Microsoft Ajax Minifier
The next option on my list of candidates was the Microsoft Ajax Minifier (ajaxmin).
Ajaxmin remains under reasonably active development and numerous blog posts show it has been actively dogfooded through internal use at Microsoft for years.
The tool is a simple stand-alone exe command line utility and installation was a breeze: Just run the downloaded .msi file and everything is put place.
The ajaxmin 'getting started' documentation shows exactly what needs to be done to run the tool, and a quick command line entry of:
ajaxmin sourcefile.js -out destfile.js
Fully minifies the code.
Hallelujah!
Less than five minutes after I had started with this tool, not only were all comments, spaces and carriage returns snipped out of the production JavaScript, all function and variable names were minified as well. All of it done right from the basic command line option and without requiring third-party libraries, platforms, wrappers or a trip down the StackOverflow rabbit hole to find an obscure command to bring that functionality about.
No extra installs. No detours to minify JavaScript variables and function names.
It just works.
But wait! There's more!
A review of the superb documentation for the ajaxmin command line switches shows a number of analysis and testing options are provided with the tool as well.
Let's try the code analysis.
Using an example where the source, destination and analysis output are all retrieved from and output to the e:\ directory, this command:
ajaxmin.exe -analyze:out e:\analysis_output.txt e:\sourcefile.js -out e:\destfile.js
Provides a listing of all actions performed on sourcefile.js to create your minified destfile.js, with that output placed in the text file analysis_output.txt
If you've ever wondered how your minifier is mapping the minified names to your functions and variables, that analysis_output.txt will give all the answers you'll need.
Any undefined global references in your code will also be flagged by that analysis, so you'll want to clean up your output by excluding any external libraries you're referencing in the code. That can be done with the -global flag, as with this example for excluding references to the jQuery library:
ajaxmin.exe -global:jQuery,$ -analyze:out e:\analysis_output.txt e:\sourcefile.js
-out e:\destfile.js
With that done, a scroll down to the bottom of your generated analysis_output.txt will point out any undeclared global references which may have escaped your earlier code reviews. It's a fantastically handy QA check.
If you need an even more extensive review of your code (and who doesn't?) you can also analyze your code with the "use strict" directive to point out any JavaScript which does not conform to the ECMA-5 standard. The -strict flag will handle that for you.
There are many other options available for further code obfuscation, such as explicit variable and property renaming, and those can be found through the ajaxmin command line switch documentation, or with use of the -? ajaxmin command line flag to display the available options. That flexibility is really nice to have, but you might not even need those more advanced options: The basic, bare-bones out-of-the box command line run of ajaxmin may provide all of the code minification you're needing.
In summary, the Microsoft Ajax Minifier provides absolutely everything needed for JavaScript code minification in a compact, clearly documented package. I cannot recommend the tool highly enough.